第一课
第一步:打开HBuilder X,新建项目。
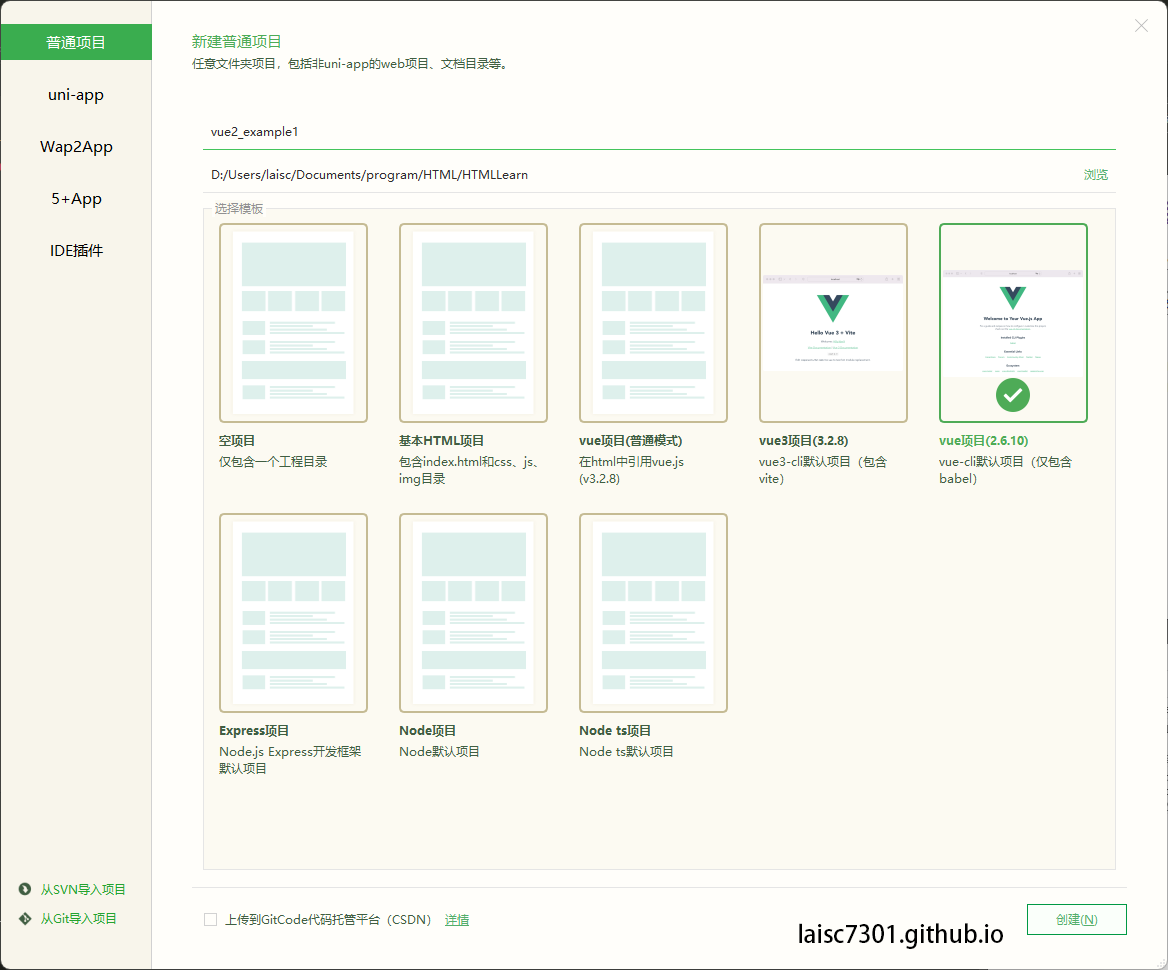
等文件下载完后,进入项目,这时如果直接运行npm run serve
,会报错,别急。
打开package.json文件,找到下面的代码:
1 2 3 4
| "scripts": { "serve": "vue-cli-service serve", "build": "vue-cli-service build" },
|
修改成这样:
1 2 3 4
| "scripts": { "serve": "SET NODE_OPTIONS=--openssl-legacy-provider && vue-cli-service serve", "build": "SET NODE_OPTIONS=--openssl-legacy-provider && vue-cli-service build" },
|
此时运行npm run serve
,就会看到欢迎页面了
现在要安装路由,在终端里运行npm install -S vue-router@3.5.3
。
现在在src里面新建一个文件,文件名为MainView.vue
。
里面输入代码:
1 2 3 4 5 6 7 8 9 10 11
| <template> <div> <router-view></router-view> </div> </template>
<script> </script>
<style> </style>
|
有几点要注意:
1.<template>
里只能有一个元素。
2.此文件是所有页面的父页面,这里输入内容会显示在所有页面上。
然后,在src目录里新建router文件夹,并在文件夹里面新建Main1.js文件,在文件里输入以下内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| import Vue from "vue" import VueRouter from "vue-router" Vue.use(VueRouter)
import MainView from "../MainView.vue"
const routes2 = [{ path: '/', redirect: '/menu', component: MainView, } ]
const router = new VueRouter({ "routes": routes2 }) export default router;
|
现在打开src目录下的main.js,修改为下面内容:
1 2 3 4 5 6 7 8 9 10 11 12
| import Vue from 'vue' import App from './MainView.vue'
import router2 from './router/Main1.js'
Vue.config.productionTip = false
new Vue({ render: h => h(App), router:router2, }).$mount('#app')
|
现在我们打开浏览器,会自动重定向到/menu页面,由于MainView.vue是所有页面的父页面,所以现在会显示MainView.vue里的内容。
第二课
现在src文件夹里新建views文件夹,在views文件夹里新建Menu.vue文件,在文件里写入以下内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <template> <div> <h1>这是一个简单的目录</h1> <h2><router-link to="/p1_main">点此进入第一个页面</router-link></h2> <h2><router-link to="/p2">点此进入Vue示例目录2</router-link></h2> <h2><router-link to="/p3">点此进入element-ui示例页面</router-link></h2> <h2><router-link to="/p4">点此进入Axios示例目录</router-link></h2> </div> </template>
<script> </script>
<style> </style>
|
打开Main1.js文件,在上面添加一行:import Menu from "../views/Menu.vue"
在routes2变量中追加:
1 2 3 4
| , { path: '/menu', component: Menu, }
|
最后Main1.js文件是这样的:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| import Vue from "vue" import VueRouter from "vue-router" Vue.use(VueRouter)
import MainView from "../MainView.vue" import Menu from "../views/Menu.vue"
const routes2 = [{ path: '/', redirect: '/menu', component: MainView, }, { path: '/menu', component: Menu, }]
const router = new VueRouter({ "routes": routes2 }) export default router;
|
现在我们可以看到一个目录页面了。
第三课
在views文件夹里新建P1_Main.vue文件,写入以下内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| <template> <div> <h1>这是一个最简单的页面</h1> <h1><router-link to="/menu">主目录</router-link></h1> <h2><router-link to="/p1_page2">点此进入下一个页面(方法1)</router-link></h2> <h2><a href="#/p1_page2">点此进入下一个页面(方法2)</a></h2>
<h2><router-link :to="{path: '/p1_page2',query: {myname: 'laisc'}}">带参数进入下一个页面(方法1)</router-link></h2> <h2><span @click="link">带参数进入下一个页面(通过js代码)</span></h2>
</div> </template>
<script> export default { name: "P1_Main", props: [], data() { return { "name": "aaa" } }, methods: { link() { this.$router.push({ path: "/p1_page2", query: { myname: 'admin' } }); } }, mounted() {
} } </script>
<style> </style>
|
在views文件夹里新建文件P1_Page2.vue,写入以下内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <template> <div id="P1_Page2">
<div>传入的名字是:{{this.$route.query.myname}}</div> </div> </template>
<script> export default { name: "P1_Page2", props: [], data() { return { "name":"aaa" } }, methods: { }, mounted() { } } </script>
<style> </style>
|
在Main1.js文件的上面添加这两行:
1 2
| import P1_Main from "../views/P1_Main.vue" import P1_Page2 from "../views/P1_Page2.vue"
|
在变量routes2中追加:
1 2 3 4 5 6 7 8
| , { path: '/p1_main', component: P1_Main, }, { path: '/p1_page2', component: P1_Page2, props: true }
|
弄完后,文件Main1.js应该是这样子:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| import Vue from "vue" import VueRouter from "vue-router" Vue.use(VueRouter)
import MainView from "../MainView.vue" import Menu from "../views/Menu.vue"
import P1_Main from "../views/P1_Main.vue" import P1_Page2 from "../views/P1_Page2.vue"
const routes2 = [{ path: '/', redirect: '/menu', component: MainView, }, { path: '/menu', component: Menu, }, { path: '/p1_main', component: P1_Main, }, { path: '/p1_page2', component: P1_Page2, props: true }]
const router = new VueRouter({ "routes": routes2 }) export default router;
|
好了,现在可以访问目录中的第一项了。
第四课
现在我们继续扩张这个项目。
找到views文件夹,在里面创建文件P2.vue,然后写入下面代码:
1 2 3 4 5 6 7 8 9 10 11
| <template> <div> <router-view></router-view> </div> </template>
<script> </script>
<style> </style>
|
然后在views文件夹里创建目录page2。
在page2文件夹里创建文件P2_Menu.vue,然后写入以下内容:
1 2 3 4 5 6 7 8 9 10 11 12 13
| <template> <div> <h1>Vue示例目录2</h1> <h2><router-link to="/menu">返回目录</router-link></h2> <h2><router-link to="/p2/p1">点此进入第一个页面</router-link></h2> </div> </template>
<script> </script>
<style> </style>
|
继续在page2文件夹里创建文件P2_P1.vue,然后写入以下内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71
| <template> <div> <h2><router-link to="/p2/menu">返回Vue示例目录2</router-link></h2> <button @click="clickme1">点我1</button> <button @click="add">点我2 - {{myNumber1}}</button> <br /> <input v-model="myInputData" />输入的内容:{{myInputData}} <br /> <span @click="changeColor" :class="bgColor=='redbg'?'redbg':'greenbg'">123456{{bgColor}}</span> <ul> <li v-for="(user, index) in jsonData1" :key="index"> {{ index + 1 }} - id={{ user.id }}, username={{ user.username }}, password={{ user.password }} </li> </ul> </div> </template>
<script> export default { name: "P2_P1", props: [], data() { return { name: "aaa", myNumber1: 0, myInputData: "", bgColor: "redbg", jsonData1: [{ "id": 1199, "username": "admin123", "password": "admin456" }, { "id": 2244, "username": "laisc", "password": "abcd" } ] } }, methods: { clickme1() { alert("我被点击了!") }, add() { this.myNumber1++; }, changeColor() { if (this.bgColor == "redbg") { this.bgColor = "greenbg"; } else { this.bgColor = "redbg"; } } }, mounted() {
} } </script>
<style> .redbg { background-color: red; }
.greenbg { background-color: green; } </style>
|
现在,打开Main1.js文件,在上面输入以下内容:
1 2 3
| import P2 from "../views/P2.vue" import P2_Menu from "../views/page2/P2_Menu.vue" import P2_P1 from "../views/page2/P2_P1.vue"
|
在变量routes2中追加:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| , { path: '/p2', redirect: '/p2/menu', component: P2, children: [{ path: 'menu', component: P2_Menu, props: true }, { path: 'p1', component: P2_P1, props: true }] }
|
最后文件Main1.js长这样:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| import Vue from "vue" import VueRouter from "vue-router" Vue.use(VueRouter)
import MainView from "../MainView.vue" import Menu from "../views/Menu.vue"
import P1_Main from "../views/P1_Main.vue" import P1_Page2 from "../views/P1_Page2.vue"
import P2 from "../views/P2.vue" import P2_Menu from "../views/page2/P2_Menu.vue" import P2_P1 from "../views/page2/P2_P1.vue"
const routes2 = [{ path: '/', redirect: '/menu', component: MainView, }, { path: '/menu', component: Menu, }, { path: '/p1_main', component: P1_Main, }, { path: '/p1_page2', component: P1_Page2, props: true }, { path: '/p2', redirect: '/p2/menu', component: P2, children: [{ path: 'menu', component: P2_Menu, props: true }, { path: 'p1', component: P2_P1, props: true }] } ]
const router = new VueRouter({ "routes": routes2 }) export default router;
|
现在目录的第二行也可以访问了。
第五课
现在要安装饿了么UI,在终端里运行npm install -S element-ui@2.9.2
然后继续。
打开main.js文件,在上面添加两行:
1 2
| import ElementUI from 'element-ui' import 'element-ui/lib/theme-chalk/index.css'
|
在中间添加一行:
最后文件长这样:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import Vue from 'vue' import App from './MainView.vue'
import router2 from './router/Main1.js'
import ElementUI from 'element-ui' import 'element-ui/lib/theme-chalk/index.css'
Vue.config.productionTip = false
Vue.use(ElementUI);
new Vue({ render: h => h(App), router:router2, }).$mount('#app')
|
在views文件夹里创建文件P3.vue,并写入以下内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74
| <template> <div> <el-menu :default-active="activeIndex" class="el-menu-demo" mode="horizontal" @select="handleSelect"> <el-menu-item index="1">处理中心</el-menu-item> <el-submenu index="2"> <template slot="title">我的工作台</template> <el-menu-item index="2-1">选项1</el-menu-item> <el-menu-item index="2-2">选项2</el-menu-item> <el-menu-item index="2-3">选项3</el-menu-item> <el-submenu index="2-4"> <template slot="title">选项4</template> <el-menu-item index="2-4-1">选项1</el-menu-item> <el-menu-item index="2-4-2">选项2</el-menu-item> <el-menu-item index="2-4-3">选项3</el-menu-item> </el-submenu> </el-submenu> <el-menu-item index="3" disabled>消息中心</el-menu-item> <el-menu-item index="p3_1">页面3_1</el-menu-item> <el-submenu index="p3_more"> <template slot="title">更多页面</template> <el-menu-item index="p3_2">页面3_2</el-menu-item> <el-menu-item index="p3_3">页面3_3</el-menu-item> </el-submenu> </el-menu> <router-view></router-view> </div> </template>
<script> export default { name: "edit", props: [], data() { return { activeIndex: '1' } }, methods: { handleSelect(key, keyPath) { console.log(key, keyPath); if (key == "p3_1") { this.$router.push({ path: "/p3/p1", query: { } }); }else if (key == "p3_2") { this.$router.push({ path: "/p3/p2", query: { } }); } else if (key == "p3_3") { this.$router.push({ path: "/p3/p3", query: { } }); } } }, mounted() {
} } </script>
<style> </style>
|
在views文件夹里创建目录page3
里面创建三个文件:
P3_P1.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <template> <div> 123 <h2><router-link to="/menu">点此进入目录</router-link></h2> </div>
</template>
<script> export default { name: "edit", props: [], data() { return { ddd:"qwer" } }, methods: { }, mounted() { } } </script>
<style> </style>
|
P3_P2.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| <template> <div> 12345 <el-button type="primary" @click="data2++;">点我吧{{data2}}</el-button> <el-button type="danger" @click="minusData3">点我吧{{data3}}</el-button> <br/> <input v-model="text123" />{{text123}} </div>
</template>
<script> export default { name: "edit", props: [], data() { return { data1: "qwer", data2: 0, data3: 100, text123:"随便输入点什么东西"
} }, methods: { minusData3() { this.data3--; }
}, mounted() {
} } </script>
<style> </style>
|
P3_P3.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72
| <template>
<table border="" cellspacing="" cellpadding=""> <tr> <th>id</th> <th>name</th> <th>price</th>
</tr> <tr v-for="item in mylist"> <td>{{item.id}}</td> <td>{{item.name}}</td> <td>{{item.price}}</td> <td><el-button type="primary">加购物车</el-button> <el-button type="success" class="myedit" id="myedit">修改</el-button> <el-button type="danger" class="mydel" id="mydel" @click="del(item.id)">删除</el-button> </td> </tr> </table> </template>
<script> export default { name: "edit", props: [], data() { return { mylist: [{ id: 1, name: "aa", price: 100.00 }, { id: 2, name: "cc", price: 200.00 }, { id: 3, name: "dd", price: 300.00 } ], }
}, methods: { del(id) { console.log(this.mylist); var newList = []; for (let i = 0; i < this.mylist.length; i++) { if (this.mylist[i].id != id) { newList.push(this.mylist[i]); } } this.mylist = newList console.log(this.mylist); }
}, mounted() {
} } </script>
<style> </style>
|
然后,打开Main1.js文件,在上面输入以下内容:
1 2 3 4
| import P3 from "../views/P3.vue" import P3_P1 from "../views/page3/P3_P1.vue" import P3_P2 from "../views/page3/P3_P2.vue" import P3_P3 from "../views/page3/P3_P3.vue"
|
在变量routes2中追加:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| , { path: '/p3', component: P3, children: [{ path: 'p1', component: P3_P1, props: true }, { path: 'p2', component: P3_P2, props: true }, { path: 'p3', component: P3_P3, props: true }] }
|
最后Main1.js文件长这样:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72
| import Vue from "vue" import VueRouter from "vue-router" Vue.use(VueRouter)
import MainView from "../MainView.vue" import Menu from "../views/Menu.vue"
import P1_Main from "../views/P1_Main.vue" import P1_Page2 from "../views/P1_Page2.vue"
import P2 from "../views/P2.vue" import P2_Menu from "../views/page2/P2_Menu.vue" import P2_P1 from "../views/page2/P2_P1.vue"
import P3 from "../views/P3.vue" import P3_P1 from "../views/page3/P3_P1.vue" import P3_P2 from "../views/page3/P3_P2.vue" import P3_P3 from "../views/page3/P3_P3.vue"
const routes2 = [{ path: '/', redirect: '/menu', component: MainView, }, { path: '/menu', component: Menu, }, { path: '/p1_main', component: P1_Main, }, { path: '/p1_page2', component: P1_Page2, props: true }, { path: '/p2', redirect: '/p2/menu', component: P2, children: [{ path: 'menu', component: P2_Menu, props: true }, { path: 'p1', component: P2_P1, props: true }] }, { path: '/p3', component: P3, children: [{ path: 'p1', component: P3_P1, props: true }, { path: 'p2', component: P3_P2, props: true }, { path: 'p3', component: P3_P3, props: true }] } ]
const router = new VueRouter({ "routes": routes2 }) export default router;
|
现在,打开浏览器,你会看到目录的第三项也可以访问了。
第六课
现在我们要安装Axios了。
在终端中输入命令:npm install -S axios@0.26.0
然后,修改main.js文件。
在文件中加入下面几行:
1 2 3 4 5
| import axios from 'axios' Vue.prototype.$axios = axios;
axios.defaults.withCredentials = true; axios.defaults.baseURL = "http://localhost:8001/";
|
弄好后文件长这样:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| import Vue from 'vue' import App from './MainView.vue'
import router2 from './router/Main1.js'
import ElementUI from 'element-ui' import 'element-ui/lib/theme-chalk/index.css'
import axios from 'axios' Vue.prototype.$axios = axios;
axios.defaults.withCredentials = true; axios.defaults.baseURL = "http://localhost:8001/";
Vue.config.productionTip = false
Vue.use(ElementUI);
new Vue({ render: h => h(App), router: router2, }).$mount('#app')
|
找到views目录,在里面添加文件:P4.vue
然后在文件中写入:
1 2 3 4 5 6 7 8 9
| <template> <div><router-view></router-view></div> </template>
<script> </script>
<style> </style>
|
在views目录中新建文件夹page4,并在page4文件夹里写入两个文件:
P4_Menu.vue
1 2 3 4 5 6 7 8 9 10 11 12 13
| <template> <div> <h1>Axios示例目录</h1> <h2><router-link to="/menu">返回目录</router-link></h2> <h2><router-link to="/p4/p1">点此进入第一个页面</router-link></h2> </div> </template>
<script> </script>
<style> </style>
|
P4_P1.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127
| <template> <div> <h2><router-link to="/p4/menu">返回Axios示例目录</router-link></h2> <button @click="get1">不带参数GET</button> {{getData1}} <br /> <button @click="get2">带参数GET</button> <button @click="post1">带参数POST</button> {{data1}} <br /> <button @click="postJson">带JSON的POST</button> <button @click="postJson2">带多个JSON的POST</button> {{data2}} <br /> <button @click="returnjson">返回JSON</button> <ul> <li v-for="(user, index) in returnjsondata" :key="index"> {{ index + 1 }} - id={{ user.id }}, username={{ user.username }}, password={{ user.password }} </li> </ul>
</div> </template>
<script> export default { name: "P4_P1", props: [], data() { return { "getData1": "默认getData1数据", "data1": "默认data1数据", "data2": "默认json数据", "returnjsondata": "" } }, methods: { get1() { //GET提交 var that = this; this.$axios.get("/test/hello", {}, {}).then( function(resp) { that.getData1 = resp.data; }) },
get2() { //GET带参数提交 var that = this; this.$axios.get("/test/test2", { params: { mydata1: "通过Get提交", a2: "ccc" } }, {}).then(function(resp) { that.data1 = resp.data; }).catch(function(error) { console.error("Error:", error); }); },
post1() { //以表单数据编码方式提交POST var that = this; this.$axios.post("/test/test2", new URLSearchParams({ mydata1: "通过Post提交", a2: "ccc" }), { headers: { 'Content-Type': 'application/x-www-form-urlencoded' //以 URL 编码的表单形式传输 }
}).then(function(resp) { that.data1 = resp.data; }); },
postJson() { //以JSON格式数据用POST提交 var that = this; this.$axios.post("/test/jsontest", { id: 111, username: "通过Post提交", password: "ccc" }, { headers: { 'Content-Type': 'application/json' }
}).then(function(resp) { that.data2 = resp.data; }); },
postJson2() { //以JSON数组格式数据用POST提交 var that = this; this.$axios.post("/test/morejsontest", [{ id: 111, username: "通过Post提交1", password: "ccc" }, { id: 222, username: "通过Post提交2", password: "ddd" }], { headers: { 'Content-Type': 'application/json' }
}).then(function(resp) { that.data2 = resp.data; }); }, returnjson() { //获得JSON数据 var that = this; this.$axios.get("/test/returnjson", {}, {}).then( function(resp) { that.returnjsondata = resp.data; }) }
}, mounted() {
} } </script>
<style> </style>
|
现在,打开Main1.js,添加这三行代码:
1 2 3
| import P4 from "../views/P4.vue" import P4_Menu from "../views/page4/P4_Menu.vue" import P4_P1 from "../views/page4/P4_P1.vue"
|
然后在变量routes2中追加:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| , { path: '/p4', redirect: '/p4/menu', component: P4, children: [{ path: 'menu', component: P4_Menu, props: true }, { path: 'p1', component: P4_P1, props: true }] }
|
最后文件长这样:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90
| import Vue from "vue" import VueRouter from "vue-router" Vue.use(VueRouter)
import MainView from "../MainView.vue" import Menu from "../views/Menu.vue"
import P1_Main from "../views/P1_Main.vue" import P1_Page2 from "../views/P1_Page2.vue"
import P2 from "../views/P2.vue" import P2_Menu from "../views/page2/P2_Menu.vue" import P2_P1 from "../views/page2/P2_P1.vue"
import P3 from "../views/P3.vue" import P3_P1 from "../views/page3/P3_P1.vue" import P3_P2 from "../views/page3/P3_P2.vue" import P3_P3 from "../views/page3/P3_P3.vue"
import P4 from "../views/P4.vue" import P4_Menu from "../views/page4/P4_Menu.vue" import P4_P1 from "../views/page4/P4_P1.vue"
const routes2 = [{ path: '/', redirect: '/menu', component: MainView, }, { path: '/menu', component: Menu, }, { path: '/p1_main', component: P1_Main, }, { path: '/p1_page2', component: P1_Page2, props: true }, { path: '/p2', redirect: '/p2/menu', component: P2, children: [{ path: 'menu', component: P2_Menu, props: true }, { path: 'p1', component: P2_P1, props: true }] }, { path: '/p3', component: P3, children: [{ path: 'p1', component: P3_P1, props: true }, { path: 'p2', component: P3_P2, props: true }, { path: 'p3', component: P3_P3, props: true }] }, { path: '/p4', redirect: '/p4/menu', component: P4, children: [{ path: 'menu', component: P4_Menu, props: true }, { path: 'p1', component: P4_P1, props: true }] } ]
const router = new VueRouter({ "routes": routes2 }) export default router;
|
现在所有事情都做完了,我们可以访问目录的最后一项了,好了,教程到此结束。
如果需要工程文件的,请发邮件获取:laisc7301@gmail.com