[!NOTE]
本教程是《SpringBoot系列基础教程》之一,教程目录:https://laisc7301.github.io/blog/2024/01/29/202401290001SpringBoot%E7%B3%BB%E5%88%97%E5%9F%BA%E7%A1%80%E6%95%99%E7%A8%8B/
整个项目的文件结构如下图所示:
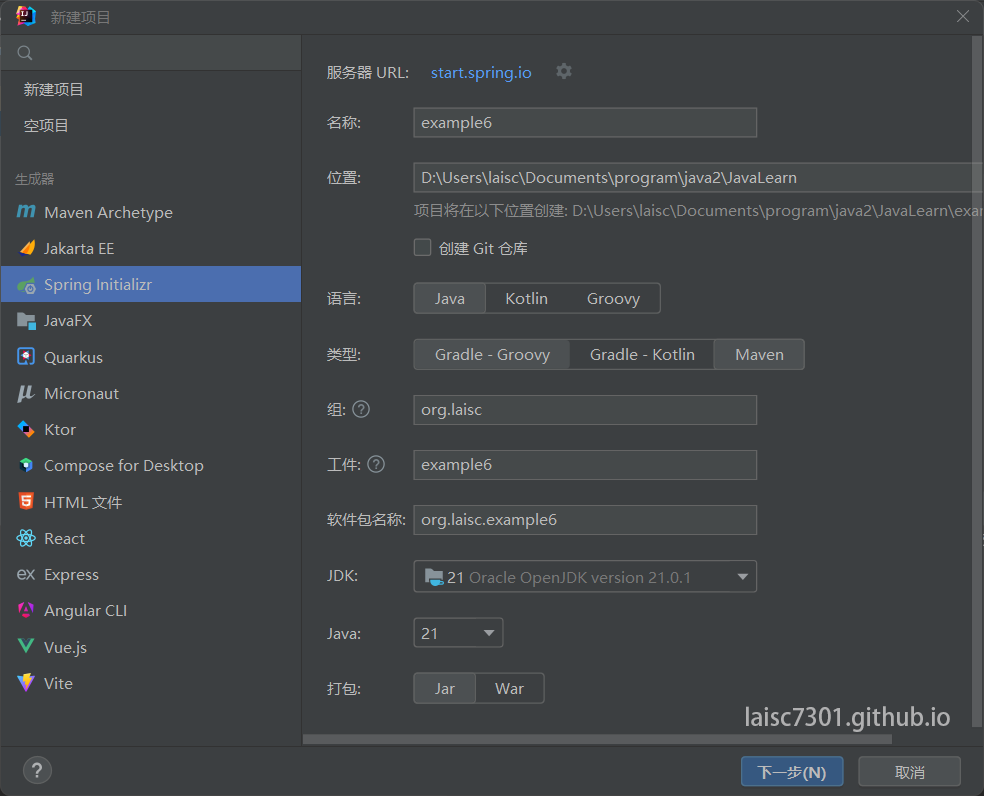
添加以下依赖:
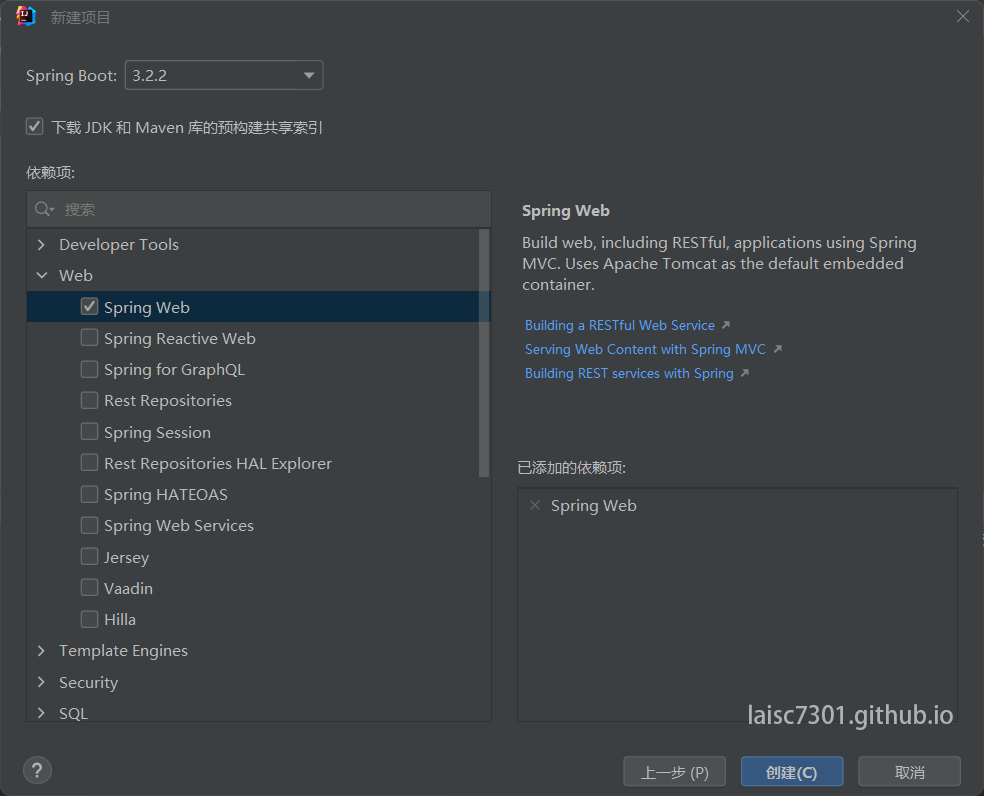
整个项目的文件结构如下图所示:
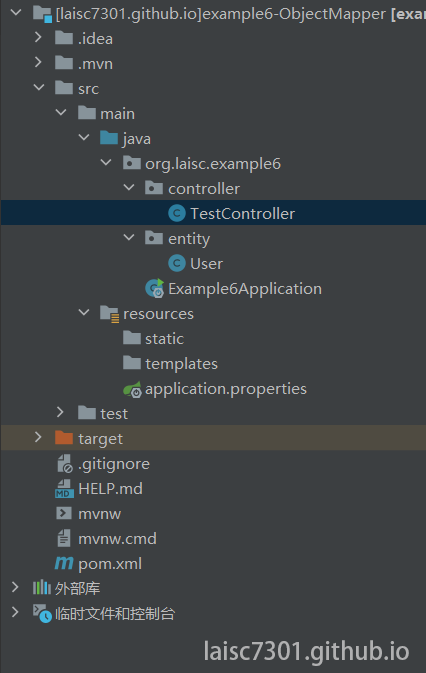
application.properties文件内容如下所示:
贴出代码,代码里的注释已经说明清楚了。
TestController.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| package org.laisc.example6.controller;
import com.fasterxml.jackson.core.JsonProcessingException; import com.fasterxml.jackson.databind.ObjectMapper; import org.laisc.example6.entity.User; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.ResponseBody;
@Controller @RequestMapping("/test") public class TestController {
@Autowired ObjectMapper objectMapper;
@ResponseBody @RequestMapping("/tojson") public String tojson() throws JsonProcessingException { User user = new User(1, "admin", "admin123","root"); String value = objectMapper.writeValueAsString(user); return value; }
@RequestMapping("/toobj") @ResponseBody public String toObj() throws JsonProcessingException { String jsonText = "{" + "\"id\": 1," + "\"username\": \"user111\"," + "\"password\": \"user1234\"," + "\"role\": \"user\"" + "}"; User u = objectMapper.readValue(jsonText, User.class); return u.toString(); } }
|
User.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| package org.laisc.example6.entity;
public class User { private Integer id = null; private String username= ""; private String password= ""; private String role= "";
public User() { }
public User(Integer id, String username, String password, String role) { this.id = id; this.username = username; this.password = password; this.role = role; }
public Integer getId() { return id; }
public void setId(Integer id) { this.id = id; }
public String getUsername() { return username; }
public void setUsername(String username) { this.username = username; }
public String getPassword() { return password; }
public void setPassword(String password) { this.password = password; }
public String getRole() { return role; }
public void setRole(String role) { this.role = role; }
@Override public String toString() { return "User{" + "id=" + id + ", username='" + username + '\'' + ", password='" + password + '\'' + ", role='" + role + '\'' + '}'; } }
|
项目源代码下载:https://pan.baidu.com/s/14t1rP8L1UvIeZtlfTMXLTQ?pwd=kvm8