[!NOTE]
本教程是《SpringBoot系列基础教程》之一,教程目录:https://laisc7301.github.io/blog/2024/01/29/202401290001SpringBoot%E7%B3%BB%E5%88%97%E5%9F%BA%E7%A1%80%E6%95%99%E7%A8%8B/
首先按照图示新建项目:
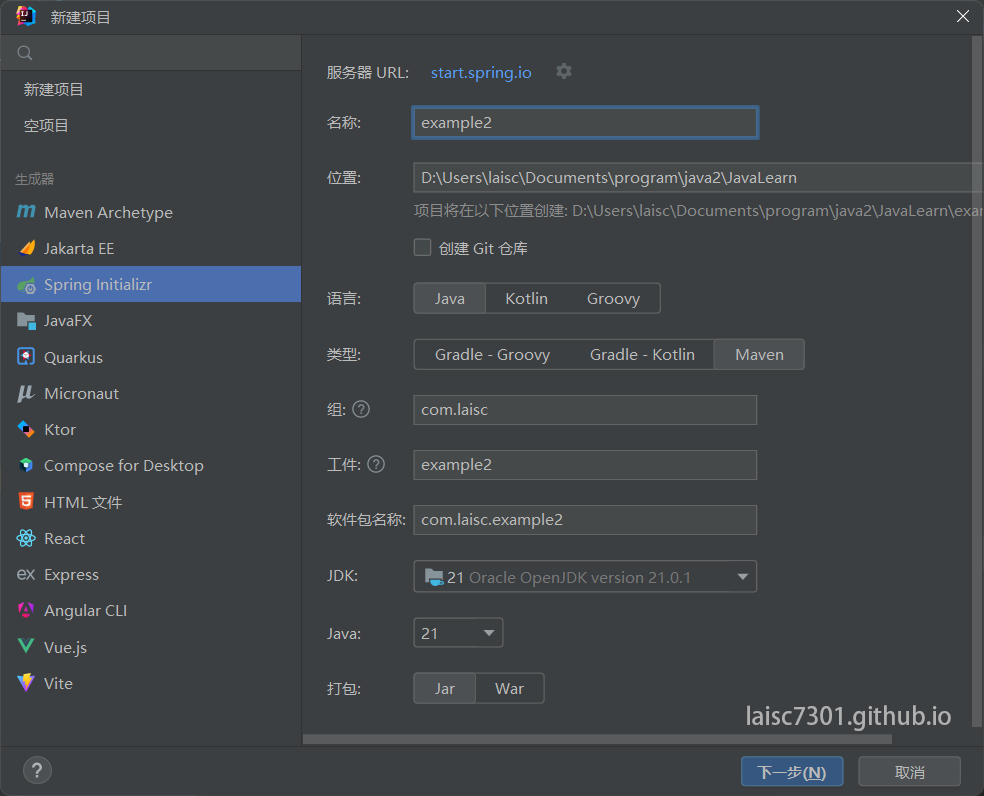
添加以下依赖:
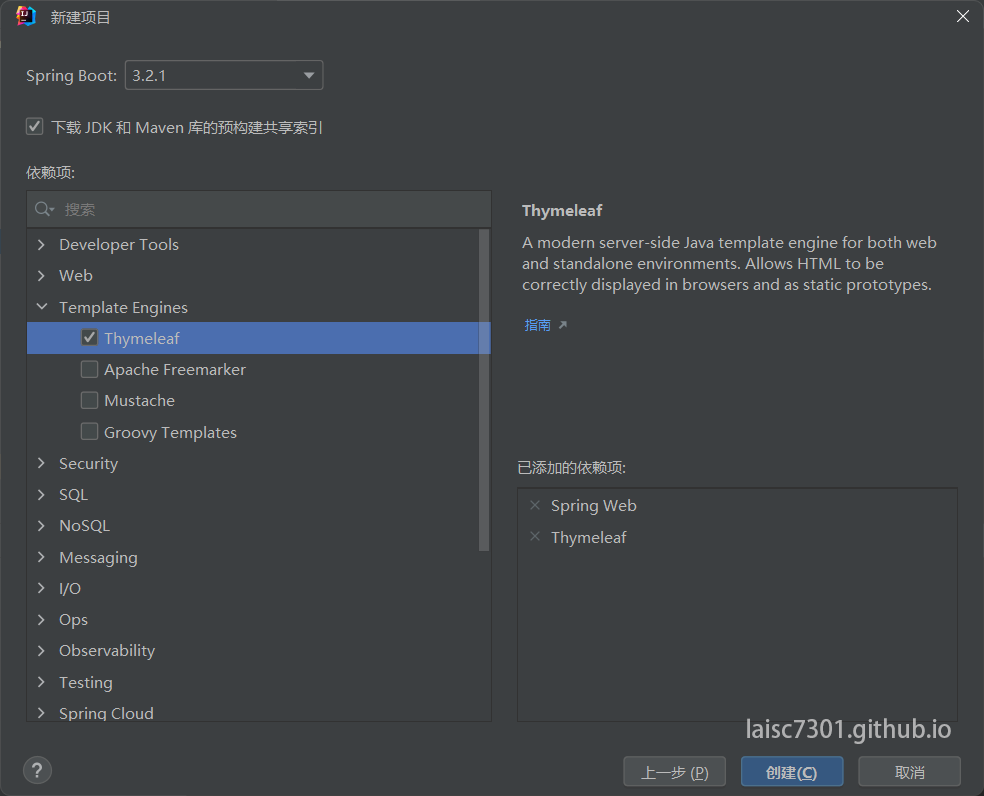
找到application.properties文件,并写入下面内容:
1 2
| server.port=8001 spring.thymeleaf.prefix=classpath:/templates/
|
如果不加spring.thymeleaf.prefix这一行,等一下会报404错误。
整个项目的文件结构如下图所示:
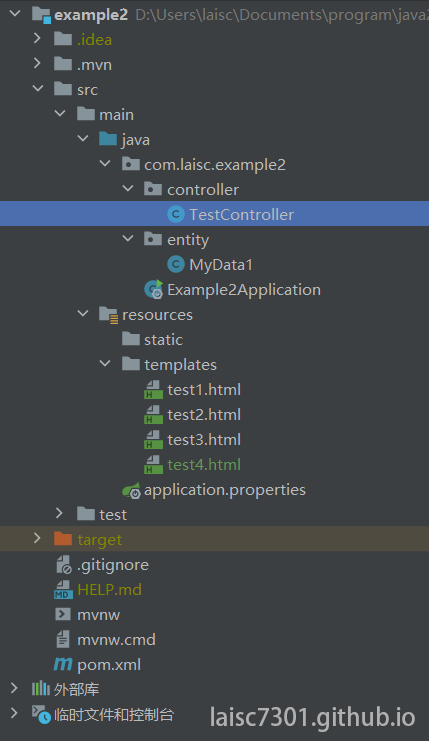
先把TestController的代码放出来:
TestController.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| package com.laisc.example2.controller;
import com.laisc.example2.entity.MyData1; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping;
import java.util.ArrayList; import java.util.List;
@Controller @RequestMapping("/test") public class TestController {
@RequestMapping("/test1") public String test1(){ return "test1"; }
@RequestMapping("/test2") public String test2(Model model){ String name = "laisc"; model.addAttribute("name", name); return "test2"; }
@RequestMapping("/test3") public String test3(Model model){ List<MyData1> myList = new ArrayList<>(); myList.add(new MyData1(1,"laisc","1234")); myList.add(new MyData1(2,"admin","qwer")); myList.add(new MyData1(3,"root","root")); model.addAttribute("list123", myList);
return "test3"; } @RequestMapping("/test4") public String test4(Model model){ MyData1 mydata1 = new MyData1(1,"laisc","1234"); model.addAttribute("data123", mydata1); return "test4"; } @RequestMapping("/test5") public String test5(HttpServletRequest request){ String name = "laisc"; request.setAttribute("name", name); return "test2"; } }
|
看这一段:
1 2 3 4
| @RequestMapping("/test1") public String test1(){ return "test1"; }
|
再看test1.html中的内容:
1 2 3 4 5 6 7 8 9 10
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> Hello, world! </body> </html>
|
这里由于return "test1";
,所以显示了test1.html的内容。
访问 http://localhost:8001/test/test1 ,你将看到:
Hello, world!
再看这一段:
1 2 3 4 5 6
| @RequestMapping("/test2") public String test2(Model model){ String name = "laisc"; model.addAttribute("name", name); return "test2"; }
|
看test2.html中的内容:
1 2 3 4 5 6 7 8 9 10 11
| <!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <p th:text="'Hello, ' + ${name} + '!'">3333</p> </body> </html>
|
此时如果访问http://localhost:8001/test/test2 ,会看到 Hello, laisc! 文字。
注意,不要漏了<html xmlns:th="http://www.thymeleaf.org">
这一行。
看这一段:
1 2 3 4 5 6 7 8 9 10
| @RequestMapping("/test3") public String test3(Model model){ List<MyData1> myList = new ArrayList<>(); myList.add(new MyData1(1,"laisc","1234")); myList.add(new MyData1(2,"admin","qwer")); myList.add(new MyData1(3,"root","root")); model.addAttribute("list123", myList);
return "test3"; }
|
再看看MyData1的代码:
MyData1.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| package com.laisc.example2.entity;
public class MyData1 { private int id = 0; private String username=""; private String password="";
public MyData1() { }
public MyData1(int id, String username, String password) { this.id = id; this.username = username; this.password = password; }
public int getId() { return id; }
public void setId(int id) { this.id = id; }
public String getUsername() { return username; }
public void setUsername(String username) { this.username = username; }
public String getPassword() { return password; }
public void setPassword(String password) { this.password = password; }
@Override public String toString() { return "MyData1{" + "id=" + id + ", username='" + username + '\'' + ", password='" + password + '\'' + '}'; } }
|
看看test3.html:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <table> <tr> <th>ID</th> <th>username</th> <th>password</th> </tr> <tr th:each="data123 : ${list123}"> <td th:text="${data123.id}">-1</td> <td th:text="${data123.username}">abc</td> <td th:text="${data123.password}">555</td> </tr> </table> </body> </html>
|
此时访问http://localhost:8001/test/test3 ,会看到下面文字。
ID |
username |
password |
1 |
laisc |
1234 |
2 |
admin |
qwer |
3 |
root |
root |
再看这一段:
1 2 3 4 5 6
| @RequestMapping("/test4") public String test4(Model model){ MyData1 mydata1 = new MyData1(1,"laisc","1234"); model.addAttribute("data123", mydata1); return "test4"; }
|
看看test4.html:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body>
<a href="details.html" th:href="@{http://localhost:8080/gtvg/order/details(orderId=${data123.username})}">链接1</a>
<a href="details.html" th:href="@{/order/details(orderId=${data123.username})}">链接2</a>
<a href="abc.html" th:href="'order/'+${data123.id}+'/details?orderId='+${data123.username} ">链接3</a>
<a href="abc.html" th:href="@{'/details/'+${data123.id}(orderId=${data123.username})}">链接4</a>
<a href="abc.html" th:href="@{'/details/'+${data123.id}+'/aaa'(orderId=${data123.username})}">链接5</a> </body> </html>
|
此时访问http://localhost:8001/test/test4 ,会看到下面几个链接。
链接1 链接2 链接3 链接4 链接5
最后看这一段:
1 2 3 4 5 6
| @RequestMapping("/test5") public String test5(HttpServletRequest request){ String name = "laisc"; request.setAttribute("name", name); return "test2"; }
|
此时如果访问http://localhost:8001/test/test5 ,会看到 Hello, laisc! 文字。
项目源代码下载:https://pan.baidu.com/s/1FK5xway6aWlTa-5hN_YlkQ?pwd=4br9
上一篇:SpringBoot入门:https://laisc7301.github.io/blog/2024/01/03/202401030000SpringBoot%E5%85%A5%E9%97%A81/
下一篇:SpringBoot使用MyBatis连接MySQL: https://laisc7301.github.io/blog/2024/01/07/202401070000SpringBoot%E4%BD%BF%E7%94%A8MyBatis%E8%BF%9E%E6%8E%A5MySQL/